What is Maven? (Explain Like I'm Five)
In short, Maven is a dependency management tool. It is perfectly normal if you don't understand anything when you first read this sentence. However, I believe that by the end of this article, you will understand this sentence. Before we start to understand what Maven is, we need to learn the concept of JAR. JAR, stands for Java Archive. It is an archive format that we can use for Java applications. The file extension for it is also .jar, as the name suggests.
Alright, but what is an archive format?
In short, it is a format that allows a Java application to be used as a single file. If you want to turn your Java application into an executable program, you convert it to Java Archive file. So, you can run your application with one command, instead of compiling and running it through your IDE (Intellij, Eclipse etc.). Â
For example, if you have methods you use frequently and don't want to rewrite them from scratch each time, you can create a project and write classes containing these methods. Then you export it as JAR file. You can import this JAR file into any project. Congrats, you made your project reusable.
In short, JAR is a tool we use to make our code independent of the IDE and reusable in other projects. When you want to reuse your project as a library, JAR comes to your help.
Ok, I understand what the JAR is, but I came here for Maven explanation!
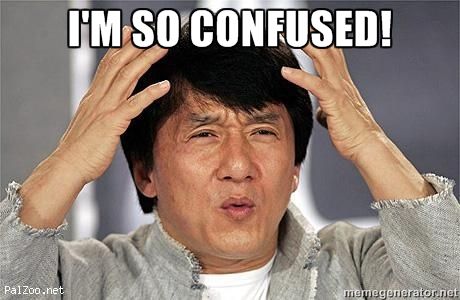
Java is a programming language that is 27 years old by the time this article is written. Therefore, programmers have faced many problems over the years. They have come up with solutions to these problems and have enabled other programmers to use these solutions. For example, there was a need to generate files in PDF format programmatically. A library was written by someone for this problem. Later, it was shared on the internet, so other programmers can use as JAR file in their projects. Libraries like these have become solutions to many problems over time. Consequently, for every problem encountered in a project, it was necessary to include a new library, a.k.a a JAR file, as a solution.
Nowadays, an enterprise Java project can easily have 100+ JAR files or dependencies in other words. Manually adding or removing these JARs one by one is not sustainable task. In addition to this, you need to go to the website of the relevant library, download the JAR file with the required version number, and manually put it into the project. As you can see, a considerable amount of time is wasted for a simple and automatable task, right? This is where Maven comes in; it automatically includes the libraries that the project depends on into the project for you.
But how does Maven do this automatically?
JAR files or in other words libraries are called dependencies in Maven. Maven keeps these dependencies in its repository. You can go to mvnrepository.com, type the name of the dependency in the search box, and find the desired dependency with its version. For example, to include the Spring Core library in our project, we need to add the following XML code to our pom.xml file.
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-core</artifactId>
<version>4.3.2.RELEASE</version>
</dependency>
The pom.xml file is Maven's configuration file. We can manage our project through this file. In short, to mention the tags briefly:
<dependency>
: Represents a dependency and contains the properties of the dependency.<groupId>
: Indicates which organization the dependency belongs to. Here, org.springframework is an organization that actually contains many libraries. In the Java world, groupId is generally related to the project's web address. If the project's web address is "blogproject.com," groupId can be "com.blogproject." This is not a requirement, just a general unwritten rule.<artifactId>
: Indicates which library will be brought in within the specified organization. For example, you can see the versions of the spring-core library from this address.<version>
: As the name suggests, it indicates which version will be brought in.
Conclusion
As I mentioned at the beginning; Maven is basically a dependency management tool. I didn't cover things like Maven installation or step-by-step usage in the article. Instead, I tried to answer questions such as what its logic is, why it is used, and what problems it solves. Of course, the capabilities Maven offers are not limited to dependency management. It also provides the ability to develop projects in a modular manner, strengthen project management with plugin support, and similar features. For more detailed information, you can visit Maven's official website.
Member discussion